Elixir’s Interactive Shell
Elixir has an interactive shell called IEx. This is a powerful and very helpful tool not only during the learning process, but when working with both development and live systems.
IEx allows to you write Elixir statements, execute them, and get the results. It has other great features like auto-completing commands (using the TAB
key), displaying help and type information.
Contents
We Learn By Doing
The most effective way to learn Elixir is to start doing Elixir. The IEx shell gives you an easy on-ramp to getting started. With Elixir installed on your system, you are ready to go.
In the coming sections, I really encourage you to play with the code as you read and learn about it. The code examples are designed to be easy to copy & paste into a terminal to make it that much easier.
Open a terminal on your computer. The command to start IEx is… iex
.
$ iex
Erlang/OTP 21 [erts-10.0.6] [source] [64-bit] [smp:4:4] [ds:4:4:10] [async-threads:1] [hipe]
Interactive Elixir (1.8.1) - press Ctrl+C to exit (type h() ENTER for help)
iex(1)>
Before we start to play, you need to know how to get out of your new play area. To exit IEx, hit CTRL+C
and you’ll see the BREAK menu:
iex(1)>
BREAK: (a)bort (c)ontinue (p)roc info (i)nfo (l)oaded
(v)ersion (k)ill (D)b-tables (d)istribution
If you press c
, you will remain in IEx. If you press a
, it will abort the shell and exit. The most common way to exit is to use CTRL+C
again. Start an IEx shell again, now hit CTRL+C
, CTRL+C
. That’s right, two times in a row. That’s the easiest way to exit an IEx shell. Commands like quit
and exit
don’t exist.
Play Time!
Now that you know how to start and exit an IEx shell, it’s time to start playing. Here are some simple commands you can try.
iex(1)> 1 + 1
2
iex(2)> b = 12
12
iex(3)> b
12
iex(4)> b + 10
22
iex(5)>
Notice that the iex
prompt includes a line number. It increments automatically. You don’t have to worry about that.
Line Continuations
When you write an expression and use an opening character that requires a closing one, it can carry across lines.
iex(1)> "abcd
...(1)> efg"
"abcd\nefg"
Notice that it included the \n
new-line character inside the string. Also note that the ...(1)>
was the continuation line.
Sometimes this happens by accident. You accidentally forget to include a matching closing character. In this example, I “forgot” to include the closing parenthesis. Eventually I figure it out, add the closing parenthesis and the function is evaluated.
iex(1)> String.downcase("HELLO WORLD"
...(1)>
...(1)>
...(1)> )
"hello world"
Auto-Complete in IEx
As mentioned, auto-complete is very handy in IEx. Time to play with it. Let’s explore that String
module that was just used to downcase some text. What else can it do? To find out, type Str
and hit your TAB
key. You should see something like this:
iex(2)> Str
Stream String StringIO
It shows modules that match the str
prefix. Add an i
and it knows you want “String” over “Stream”. Hit TAB
and let it complete. Now add a .
and hit TAB
again to see all the modules and functions available under the String
module. It should look something like this:
iex(2)> String.
Break Casing Chars
Tokenizer Unicode at/2
bag_distance/2 capitalize/1 capitalize/2
chunk/2 codepoints/1 contains?/2
downcase/1 downcase/2 duplicate/2
ends_with?/2 equivalent?/2 first/1
graphemes/1 jaro_distance/2 last/1
length/1 match?/2 myers_difference/2
next_codepoint/1 next_grapheme/1 next_grapheme_size/1
normalize/2 pad_leading/2 pad_leading/3
pad_trailing/2 pad_trailing/3 printable?/1
printable?/2 replace/3 replace/4
replace_leading/3 replace_prefix/3 replace_suffix/3
replace_trailing/3 reverse/1 slice/2
slice/3 split/1 split/2
split/3 split_at/2 splitter/2
splitter/3 starts_with?/2 to_atom/1
to_charlist/1 to_existing_atom/1 to_float/1
to_integer/1 to_integer/2 trim/1
trim/2 trim_leading/1 trim_leading/2
trim_trailing/1 trim_trailing/2 upcase/1
upcase/2 valid?/1
iex(2)> String.
I see “length/1”. That seems obvious enough. I expect it will return the length of a string. Let’s try it.
iex(2)> String.length("elixir")
6
It returned the number of characters in the string. Note: the /1
means the function takes 1 argument.
Help in IEx
As mentioned before, IEx has the ability to get “help” on modules, functions and more. Let’s see the help for String.length
. The help is displayed by putting the h
command in front of a module name or a module and function name together as in the following example:
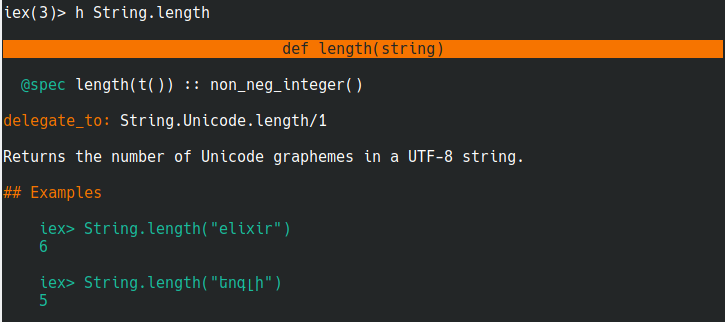
You will notice that this help text is the exact same as what is in the online documentation. String.length/1 documentation. This is no coincidence. The documentation is generated from the help text included in the code. Likewise, your code always has the documentation for the exact version of Elixir that you are running. That’s pretty cool! Still works when you are on an airplane.
Command History
Being able to press the UP
arrow key and bring back the last command can be really helpful. When you start IEx, you can pass in a command to make that available like this:
$ iex --erl "-kernel shell_history enabled"
This command is passing an Erlang configuration option through IEx using --erl
. The command will enable shell history. Now, when in an IEx shell, pressing the UP
arrow brings back the previous command. Hitting the UP
arrow repeatedly lets you keep going back through the command history.
Entering that command every time you start IEx isn’t fun. You can set the ERL_AFLAGS
environment variable on your system through your shell’s profile file to make it always available.
On Unix-like / Bash: (ie: MacOS/Linux)
export ERL_AFLAGS="-kernel shell_history enabled"
On Windows:
export ERL_AFLAGS="-kernel shell_history enabled"
On Windows 10 / PowerShell:
$env:ERL_AFLAGS = "-kernel shell_history enabled"
Don’t worry about doing this right now if it’s not important to you at the moment. Just know that it can be solved and you have the solution right here when you are ready for it.
Ready, Set, Go!
With Elixir installed and the ability to play in the interactive IEx shell, you are ready to go! Remember that play is fun. So now it’s time to have fun!
6 Comments
Leave a Comment
You must be logged in to post a comment.
I’m wondering is it possible to change colours/theme of iex, like in zsh/bash we have oh-my-zsh. I suppose it’s impossible but I noticed that in different terminals iex looks different.
👏 The lesson was useful for me. I didn’t know about kernel shell_history though I used iex previously.
Create a new file named “.iex.exs” in your current directory. Add the following contents, save the file, then open iex…Note: I learned this from Dave Thomas.
Awesome! Thanks for sharing!
Two notes for those who care:
1. When using Windows Powershell, one must invoke iex using “iex.bat”
2. If you want the auto-complete, then you must do “iex.bat –werl”, which will launch the Erlang iex, which then allows the Tab-based auto-complete.
Came here for this comment. Thanks!